Dynamics AX 2012 Coding Best Practices
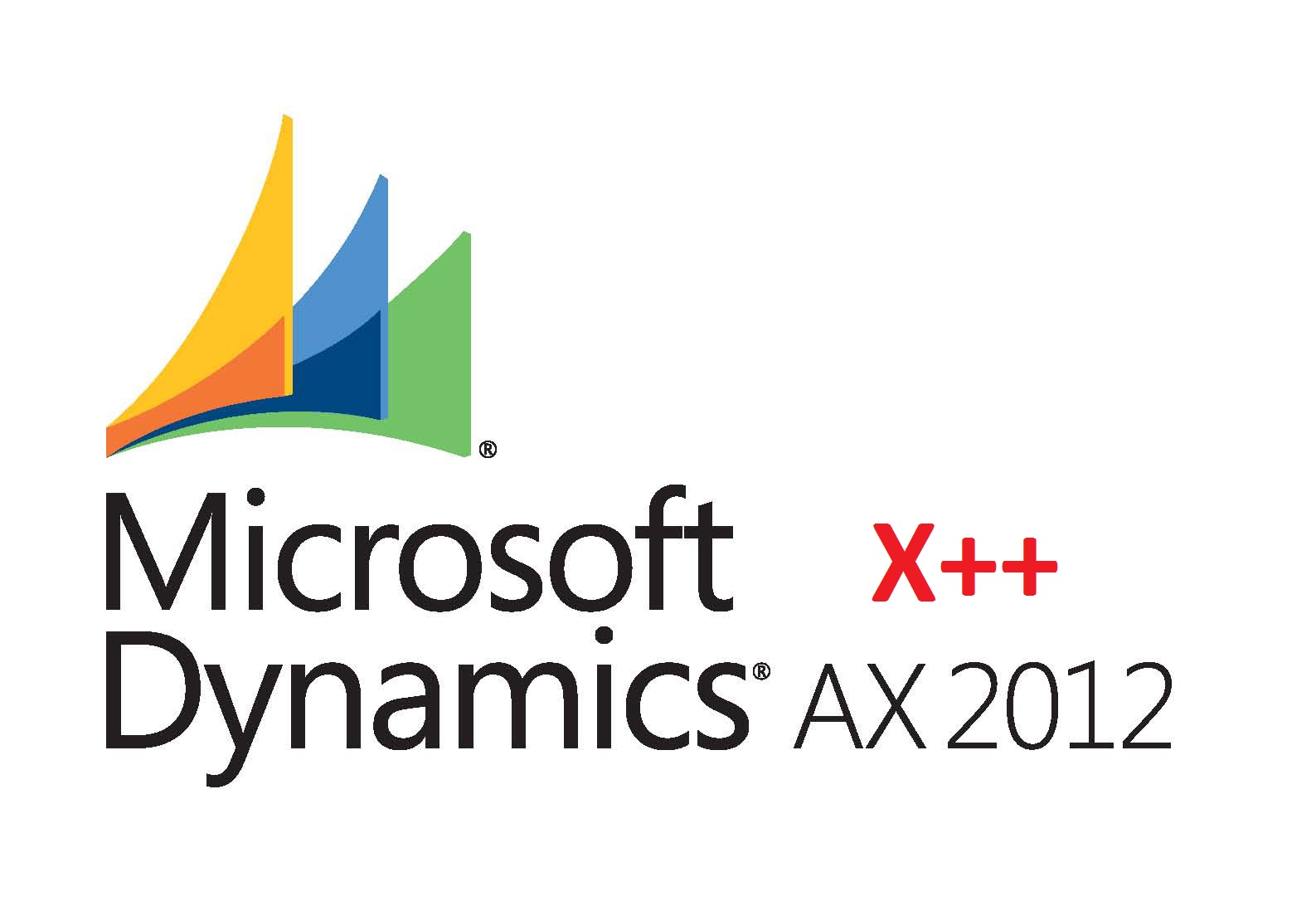
23 Jan 2015
Hi Guys,
In this post I’ll be talking about Dynamics AX coding best practices i.e. the best practices that you should follow when using X++ code in Dynamics AX 2012.
Coding in AX involves a number of items from naming conventions to select and conditional statements, the use of comments, semicolons, transactions, etc. and there are best practices associated with the use of each of these items, if you want to create the perfect piece of code that offers great performance and is also easy to debug. Let’s take a look at some of these best practices below.
Dynamics AX 2012 Coding Best practices for Naming Conventions
- When using naming conventions, wherever possible try to ensure that your application object names are constructed hierarchically from these 3 basic components: {business area name} + {business area description} + {action performed (for classes) or type of contents (for tables)}. For example, CustInvoicePrintout, PriceDiscAdmCopy
- Try to ensure that your application objects names are mixed case, with the first letter of the application object being uppercase and the first letter of each internal word also in uppercase. These application object naming convention applies to tables, maps, extended data types, base enums, table collections, macros, classes, forms, reports, queries, menus, and menu items. For example, AddressFormatHeading, SalesAmount
- Try to use mixed-case names for methods, system functions, and variables, with a lowercase first letter and the first letter of each internal word capitalized. Like for classDeclaration, createProject
- Use lowercase names for Primitive data types
For more details on naming convention best practices, have a look at this link.
Dynamics AX 2012 Coding Best practices for Select Statements
- When using Select statements be sure to indent the index, order by and where statements relative to the Select or While Select statement you’re using.
- Make sure that the Where expression is structured in a column.
- Ensure that any Boolean operators (&&) used are at the beginning of the line, and in columns
- Make sure that the While Select block has braces, even if it contains only one statement
- Also try and keep the braces at the same column position as the While block
- Make sure that uppercase and lowercase name standards are adhered to
Dynamics AX 2012 Coding Best practices for Comments
- To find comments in the source code (both // .. and /* .. */), use the Find dialog to search for methods containing the text (regular expression): /[/*]
- Comments should not include:
- Dates
- Names
- Aliases
- Version or layer references
- Bug numbers (unless it is a workaround, or unless the code will appear inappropriate if you don’t know that it was for a bug fix.
- Politically or culturally sensitive phrases
- If you’re putting a comment at the start of the method to describe its purpose and use, then you should use block comments (/* */)
Dynamics AX 2012 Coding Best practices for Using Extra Semicolons
- From Microsoft Dynamics AX 2012 onward, it’s not mandatory to put a semicolon on an empty line after the last variable declaration.
Dynamics AX 2012 Coding Best practices for Using Constants
When using Constants try to use intrinsic functions whenever possible. Intrinsic functions are metadata assertion functions. They take arguments that represent entities in the Application Object Tree (AOT) and validate these arguments at compile time. Learn more
Dynamics AX 2012 Coding Best practices for User interface labels
- When using User Interface labels, make sure to use complete sentences. Also, do not build sentences using more than one label, or other constants or variables under program control (don’t use concatenation). For example:
Description description = “@SYS12345”
Use strFmt to format user interface text.
Dynamics AX 2012 Coding Best practices for System Oriented Constants
- Do not use labels since you’ll get a warning if a label is used inside single quotes.
- When using Dates:
- Use only strongly typed (date) fields, variables and controls (do not use str or int).
- Use Auto settings in date formatting properties.
- Use DateTimeUtil::getSystemDateTime instead of systemDateGet or today
- Avoid using date2str for date conversions.
- Your application logic should use the system function systemDateGet which holds the system’s date (this can be set from the status bar).
- Only use the system function today() when the actual machine date is needed
- Use strFmt or date2Str with -1 in all formatting parameters. This will ensure that the date is formatted in the way that the user has specified in their Regional Settings
Dynamics AX 2012 Coding Best practices when using the Try catch Block
- Always create a try/catch deadlock/retry loop around database transactions that you believe may potentially lead to deadlocks
- Whenever you have a retry call, all the transient variables must be set back to the value they had just before the try. The persistent variables (i.e. the database and the Infolog) are set back automatically by the throw that leads to the catch/retry. For example:
try
{
this.createJournal();
this.printPosted();
}
catch (Exception::Deadlock)
{
this.removeJournalFromList();
retry;
}
As you can see from the sample code above, the throw statement automatically initiates a ttsAbort, which is a database transaction rollback.
- The throw statement should only be used if a piece of code cannot do what it is expected to do. It should not be used for more ordinary program flow control.
Dynamics AX 2012 Coding Best practices when using Transactions
- The ttsBegin and ttsCommit commands must always be used in a clear and well-balanced manner. Balanced ttsBegin and ttsCommit statements are:
- Always in the same method and
- Always on the same level in the code
- Avoid making only one of the ttsBegin or ttsCommit commands conditional
- Use the throw statement if a transaction cannot be completed.
- Don’t use ttsAbort, use the throw statement instead.
Dynamics AX 2012 Coding Best practices when using Conditional Statements
- When using Conditional statements, if you have an if…else construction, then use positive logic. Avoid using:
if (!false)
{
…
}
Dynamics AX 2012 Coding Best practices in XML Documentation
The following table lists the best practices when dealing with error messages and resolving them.
Message | Message type | How to fix the error or warning |
Tag ‘%1’ in XML documentation is not supported. | Warning | Add XML documentation. For information about how to add XML documentation, see How to: Add XML Documentation to X++ Source Code. Because this is a warning instead of an error, this is optional. |
Unsupported tag ‘%1’ in XML documentation. | Error or Warning | Check the casing of the XML tags if this is reported as an error. If this is reported as a warning, an unsupported tag is present. Remove unsupported tags. |
Missing tag ‘<param name=”%1″>’ in XML documentation. | Error | Add <param> tags to the XML header template. The <param> tag must have a name attribute. The value of the attribute is case-sensitive and must match the casing in the method. |
Missing tag ‘returns’ in XML documentation. | Error | Add <returns> tags to the XML header template. |
Missing tag ‘summary’ in XML documentation. | Error | Add <summary> tags to the XML header template. |
Tag ‘%1’ exists more than once in XML documentation. | Error | Delete extra tags. This applies only when multiple tags are not appropriate. |
Tag ‘<param name=”%1″>’ has no content in XML documentation. | Error | Add a description of the parameter between the <param> tags. |
Tag ‘<param name=”%1″>’ in XML documentation doesn’t match actual implementation. | Error | Fix the value of the name attribute. It is case-sensitive and must match the casing in the method. |
Tag ‘exception’ has no content in XML documentation. | Error | Add a description of the exception between the <exception> tags. |
Tag ‘permission’ has no content in XML documentation. | Error | Add a description of the required permission between the <permission> tags. |
Tag ‘remarks’ has no content in XML documentation. | Error | Add content between the <remarks> tags or delete the <remarks> tags. |
Tag ‘returns’ has no content in XML documentation. | Error | Add a description of the return value between the <returns> tags. |
Tag ‘returns’ in XML documentation doesn’t match actual implementation. | Error | Delete the <returns> tags and the description of the return value. |
Tag ‘summary’ has no content in XML documentation. | Error | Add a topic summary between the <summary> tags. |
XML documentation is not well-formed. | Error | Make sure that there are no mistakes in the XML tags. Each opening tag must have a corresponding closing tag. |
Tag ‘seealso’ has no content in XML documentation. | Error | Add content between the <seealso> tags or delete the <seealso> tags. |
No XML documentation for this method. | Error | XML documentation has not been written for this method. |
Avoiding Potential Security Issues
- Certain APIs that ship with Microsoft Dynamics AX use Code Access Security. When these APIs are run on the server, a class derived from CodeAccessPermission must be used. This helps make the APIs more secure.
- When you upgrade from a previous version of Microsoft Dynamics AX, you should modify the calls to these APIs, in order to ensure that the code executes correctly. details
Other Dynamics AX 2012 Coding Best practices
For more Dynamics AX 2012 Coding Best practices try this link
References
About Folio3 Dynamics Services
FDS (Folio3 Dynamics Services) is a dedicated division of Folio3 that specializes in Microsoft Dynamics AX, Dynamics CRM, Dynamics NAV and Dynamics GP based development, customization and integration services. Our main focus is on Enterprise Mobility. We firmly believe that opening up Microsoft Dynamics AX and other products to iOS Android, Windows 8 and Windows Phone users will have a huge impact on organizational agility and productivity. If you have a Dynamics AX development requirement you would like to discuss or would like to know more about our Dynamics AX development services, please get in touch with us.